概要
IDW(Inverse Distance Weighted)法とは、逆距離加重法ともいわれ、評価点に近いほど、平均化処理への影響が大きくなります。距離の逆数を重みとした加重平均で値を求めることができます。
内容
Zpを補間値、ziを各点の値、wiを評価点と各点の距離の逆数とすると、C#では以下のように記述することができます。
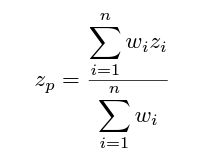
using System;
class TestClass
{
static void Main(string[] args)
{
Interpolation inter = new Interpolation();
//補間値
double v = inter.calc();
}
}
public class Interpolation
{
//座標
double[,] _coord = new double[,] { { 0, 0 }, { 0, 4 }, { 3.4, 2 } };
//値
double[] _value = new double[] { 100, 200, 300 };
//求めたい座標
double[] _target;
public Interpolation()
{
_target = new double[] { 0.0, 0.0 };
//求めたい座標
_target[0] = 2.0;
_target[1] = 2.5;
}
public double calc()
{
double number = 0.0;
double denom = 0.0;
for (int i= 0; i < _value.Length; i++)
{
double w = W(i);
number += w * _value[i];
denom += w;
}
return number / denom;
}
public double W(int i)
{
double value = Math.Sqrt(
(_target[0] - _coord[i, 0]) * (_target[0] - _coord[i, 0])
+ (_target[1] - _coord[i, 1]) * (_target[1] - _coord[i, 1]));
//同一座標の場合
if(value == 0.0)
{
return 10000000;
}
return 1.0 / value;
}
}