AjaxでHTMLメッセージを出力(dataType = html)
概要
以下のように画面を作成します。
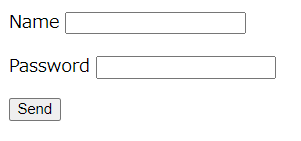
Sendボタンをクリックすると、サーバー側でパメラータのメッセージを返します。通信はajaxを使っています。(jquery)
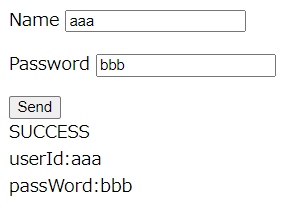
htmlサイド
index.htmlは、以下の通りです。
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>PHP Ajax Sample</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<form id="form_sample" method="post">
<p>Name <input type="text" name="userid" id ="userid"></p>
<p>Password <input type="text" name="password" id="password"></p>
</form>
<button id="ajax">Send</button>
<div class="result"></div>
<script>
$(function() {
$('#ajax').on('click', function() {
$.ajax({
url: './ajax1.php',
type: 'POST',
data: {
'userid':$('#userid').val(),
'password':$('#password').val()
},
dataType: 'html',// "text" or "html" or "xml" or "script" or "json" or "jsonp"
})
.done((response) => {
$('.result').html(response);
console.log(response);
})
.fail((response) => {
$('.result').html(response);
console.log(response);
})
.always((response) => {
});
});
});
</script>
</body>
</html>
PHPサイド
ajax1.phpは、以下の通りです。
<?php
header('Content-type: text/plain; charset=UTF-8');
if (isset($_POST['userid']) && isset($_POST['password'])) {
$id = $_POST['userid'];
$pass = $_POST['password'];
$str = "SUCCESS\nuserId:".$id."\npassWord:".$pass."\n";
$result = nl2br($str);
echo $result;
} else {
echo "FAIL";
}
Ajaxでメッセージを出力(dataType = text)
概要
以下のように画面を作成します。
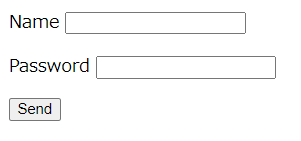
Sendボタンをクリックすると、サーバー側でパメラータのメッセージを返します。通信はajaxを使っています。(jquery)
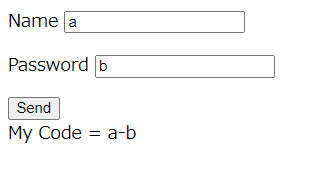
htmlサイド
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>PHP Ajax Sample</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<form id="form_sample" method="post">
<p>Name <input type="text" name="userid" id ="userid"></p>
<p>Password <input type="text" name="password" id="password"></p>
</form>
<button id="ajax">Send</button>
<div class="result"></div>
<script>
$(function() {
$('#ajax').on('click', function() {
$.ajax({
url: './ajax1.php',
type: 'POST',
data: {
'userid':$('#userid').val(),
'password':$('#password').val()
},
dataType: 'text',
})
.done((response) => {
var messge = response;
$('.result').html(messge);
console.log(response);
})
.fail((response) => {
$('.result').html(response);
console.log(response);
})
.always((response) => {
});
});
});
</script>
</body>
</html>
PHPサイド
<?php
header('Content-type: text/plain; charset=UTF-8');
if (isset($_POST['userid']) && isset($_POST['password'])) {
$id = $_POST['userid'];
$pass = $_POST['password'];
$result = "My Code = ".$id."-".$pass;
echo $result;
} else {
echo "FAIL";
}
パスワードを入力してファイルをダウンロード
概要
以下のように画面を作成します。
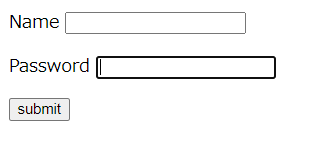
Sendボタンをクリックすると、a.dxfファイルをダウンロードします。
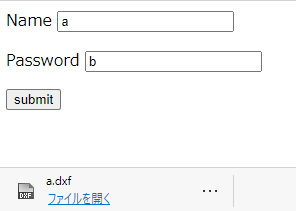
htmlサイド
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>PHP Ajax Sample</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<form id="form_sample" action="./ajax1.php" method="post">
<p>Name <input type="text" name="userid" id ="userid"></p>
<p>Password <input type="text" name="password" id="password"></p>
<input type="submit" value="submit">
</form>
</body>
</html>
PHPサイド
<?php
$dxf = "version 1"."\n";
$file_name = "a.dxf";
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename*=UTF-8\'\''.rawurlencode($file_name));
echo $dxf;
?>
Ajaxでファイルをダウンロード
概要
以下のように画面を作成します。
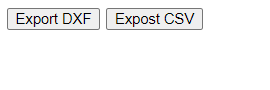
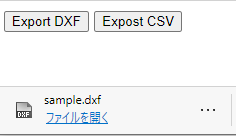
“Export DXF”をクリックすると、sample.dxfがダウンロードできます。”export_CSV”の方は、未実装です。
htmlサイド
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>PHP Ajax Sample</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<div id="info">
<button id="export_DXF" class="button">Export DXF</button>
<button id="export_CSV" class="button">Expost CSV</button>
</div>
<script>
$(function() {
$('#export_DXF').on('click', function() {
$.ajax({
url: './ajax1.php',
type: 'POST',
data: {
'userid':$('#userid').val(),
'password':$('#password').val()
},
dataType: 'text',
})
.done((response) => {
var messge = response;
export_DXF(messge);
console.log(response);
})
.fail((response) => {
$('.result').html(response);
console.log(response);
})
.always((response) => {
});
});
});
function export_DXF (data){
var blob = new Blob([data], {type: "text/plain"});
var a = document.createElement("a");
a.href = URL.createObjectURL(blob);
a.target = '_blank';
a.download = 'sample.dxf';
a.click();
}
</script>
</body>
</html>
PHPサイド
<?php
$dxf = "version 1"."\n";
echo $dxf;
?>